728x90
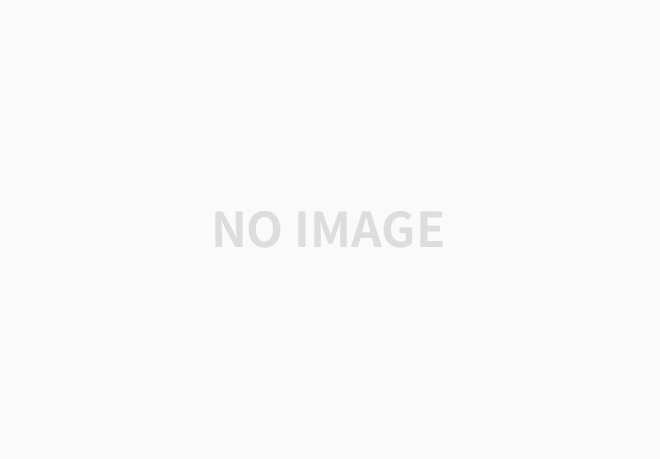
이전 방식과는 다르게 풀어보려고 했다가,, 머리가 굳어서인지 된통 당했다.
1. 낚시꾼을 한칸씩 움직인다.
2. 상어들을 움직인다.
3. 한놈씩 잡아낸다.
상어를 움직일 때 좌표변환만 유의하면 특별히 어렵지 않은 문제이다.
17143번: 낚시왕
낚시왕이 상어 낚시를 하는 곳은 크기가 R×C인 격자판으로 나타낼 수 있다. 격자판의 각 칸은 (r, c)로 나타낼 수 있다. r은 행, c는 열이고, (R, C)는 아래 그림에서 가장 오른쪽 아래에 있는 칸이다.
www.acmicpc.net
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.Collections;
import java.util.StringTokenizer;
public class Main {
static BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
static StringTokenizer st;
static int r,c,m,ans;
static Shark[][] map,mmap;
static boolean[][] check;
static int[] dy = {0,-1, 1, 0, 0}; // 상하우좌
static int[] dx = {0, 0, 0, 1,-1};
static ArrayList<Shark> list = new ArrayList<Shark>();
static class Shark implements Comparable<Shark>{
int s,d,z,y,x;
public Shark(int s, int d, int z, int y, int x) {
this.s = s;
this.d = d;
this.z = z;
this.y = y;
this.x = x;
}
@Override
public int compareTo(Shark o) {
return (this.z-o.z);
}
}
private static void solution() {
for(int fisherman=0; fisherman<c; fisherman++) {
for(int line=0; line<r; line++) {
if(map[line][fisherman]!=null) { // 1. 상어가 있으면 잡고
ans += map[line][fisherman].z;
map[line][fisherman] = null; // 2. 상어 지우고
break;
}
}
allSharksMove(); // 3. 남은 상어들 움직인다.
}
}
private static void allSharksMove(){
mmap = new Shark[r][c];
for(int i=0; i<r; i++) {
for(int j=0; j<c; j++) {
if(map[i][j]!=null) {
list.add(new Shark(map[i][j].s,map[i][j].d,map[i][j].z, i, j)); // 상어 한마리씩 더함
}
}
}
Collections.sort(list);
for(Shark s : list) {
oneSharkMove(s,s.y,s.x);
}
list.clear();
map = mmap;
}
private static void oneSharkMove(Shark old, int y, int x) {
// 움직일 좌표 결정
int spd = old.s;
int dir = old.d;
int spdY = spd%((r-1)*2);
int spdX = spd%((c-1)*2);
int ny = y;
int nx = x;
if(dir==1 || dir==2) {
for(int i=0; i<spdY; i++) {
ny += dy[dir];
if(ny<0) {
ny+=2;
dir=2;
}else if(ny>r-1) {
ny-=2;
dir=1;
}
}
}else {
for(int i=0; i<spdX; i++) {
nx += dx[dir];
if(nx<0) {
nx+=2;
dir=3;
}else if(nx>c-1) {
nx-=2;
dir=4;
}
}
}
mmap[ny][nx]= new Shark(spd,dir,old.z,ny,nx);
}
public static void main(String[] args) throws IOException {
st = new StringTokenizer(br.readLine());
r = Integer.parseInt(st.nextToken());
c = Integer.parseInt(st.nextToken());
m = Integer.parseInt(st.nextToken());
map = new Shark[r][c];
for(int i=0; i<m; i++) {
st = new StringTokenizer(br.readLine());
int y = Integer.parseInt(st.nextToken())-1;
int x = Integer.parseInt(st.nextToken())-1;
int ss = Integer.parseInt(st.nextToken());
int dd = Integer.parseInt(st.nextToken());
int zz = Integer.parseInt(st.nextToken());
map[y][x] = new Shark(ss,dd,zz,y,x); // 상어 상태 입력
}
solution();
System.out.println(ans);
}
}
728x90
'[Algorithms] > [Problems]' 카테고리의 다른 글
BOJ. 2638번. 치즈 (0) | 2021.03.19 |
---|---|
BOJ. 11559번. Puyo Puyo (0) | 2021.03.17 |
BOJ. 19236번. 청소년상어 (0) | 2021.03.15 |
BOJ. 19237번. 어른상어 (0) | 2021.03.14 |
BOJ. 16236번. 아기상어 (0) | 2021.03.14 |